Its now time to start with our first entrant into the ORM throwdown: NHibernate. I’ll spare you the nonsense about what NHibernate is and why you should use it. For now, I’ll just point you to nhforge.org for more information about the tool.
As a good practice, I prefer to isolate my NHibernate implementation of the data access layer in a class library project. This implementation allows me to upgrade and tune the data access layer without directly modifying the rest of the application. Others will argue that by doing this, I have lost the ability to control the boundaries of a unit of work in the context of a web session. While I agree, I have given up that control, I prefer to have the isolation of my data access code.
First thing to build is a ProductRepository that will access the database. To do this, with NHibernate, we need an XML configuration file that will define the dialect NHibernate will generate and utilize when communicating with our data store. My hibernate.cfg.xml
file looks like this:
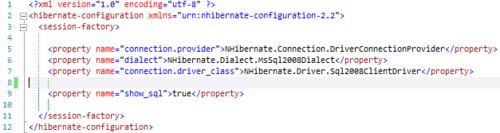
We next need to build an object that will facilitate the presentation of NHibernate sessions. The NHibernate session is our gateway to the database. The catch here is, the session factory initialization is a performance drain. To combat this, I have created a simple (read: not optimized) wrapper to provide static access to the factory:
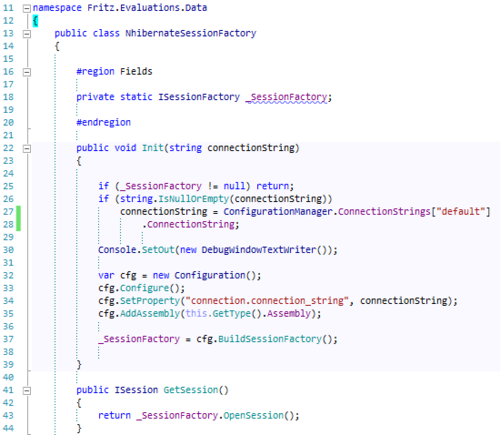
A quick note: see line 30.. I have written a TextWriter that will output to the immediate window in VisualStudio every sql statement that NHibernate compiles. Yeah, I’m tricky like that 😉
I have written a class, therefore its time to write a test:

I have placed my sql connection string in a constant in this class, hidden in the Fields region, and marked the test as ‘Ignore’. Why? I don’t want to run this very time-consuming unit test every time I run my suite, this is more a verification that we are configured properly.
Next steps are to create concrete IEvaluation and IProduct objects, and create a ProductRepository. I won’t bore you with the concrete object definitions and mapping files here, but the repository to use these objects looks something like:
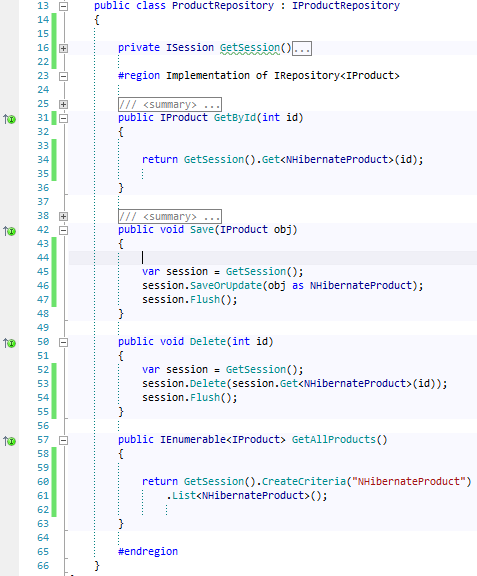
NDepend reports the following on our NHibernate project:
56 lines of C# code across 5 classes. The most complex method (SessionFactory.Init) has a cyclomatic complexity of 3. All other methods have a complexity of 1. This is good… it means the code is not complex.
However, there is a distinct code smell, as direct references to the database structure are now embedded in my DLL. Additionally, all of these references are buried in object mapping hbm.xml files that are embedded resources in the DLL… This library is NOT very resistant to changes in the database without modifications and recompiling.
Source code is now available at: https://github.com/csharpfritz/Fritz.Evaluations
In our next post we’ll look at Microsoft’s offering in the ORM game – THE Entity Framework.